1.线程等待策略
在base/wait_strategy.h
这个头文件中定义了线程切换的策略类:
class WaitStrategy { public: virtual void NotifyOne() {} virtual void BreakAllWait() {} virtual bool EmptyWait() = 0; virtual ~WaitStrategy() {} };
class BlockWaitStrategy : public WaitStrategy { public: BlockWaitStrategy() {} void NotifyOne() override { cv_.notify_one(); }
bool EmptyWait() override { std::unique_lock<std::mutex> lock(mutex_); cv_.wait(lock); return true; }
void BreakAllWait() override { cv_.notify_all(); }
private: std::mutex mutex_; std::condition_variable cv_; };
class SleepWaitStrategy : public WaitStrategy { public: SleepWaitStrategy() {} explicit SleepWaitStrategy(uint64_t sleep_time_us) : sleep_time_us_(sleep_time_us) {}
bool EmptyWait() override { std::this_thread::sleep_for(std::chrono::microseconds(sleep_time_us_)); return true; }
void SetSleepTimeMicroSeconds(uint64_t sleep_time_us) { sleep_time_us_ = sleep_time_us; }
private: uint64_t sleep_time_us_ = 10000; };
class YieldWaitStrategy : public WaitStrategy { public: YieldWaitStrategy() {} bool EmptyWait() override { std::this_thread::yield(); return true; } };
class BusySpinWaitStrategy : public WaitStrategy { public: BusySpinWaitStrategy() {} bool EmptyWait() override { return true; } };
class TimeoutBlockWaitStrategy : public WaitStrategy { public: TimeoutBlockWaitStrategy() {} explicit TimeoutBlockWaitStrategy(uint64_t timeout) : time_out_(std::chrono::milliseconds(timeout)) {}
void NotifyOne() override { cv_.notify_one(); }
bool EmptyWait() override { std::unique_lock<std::mutex> lock(mutex_); if (cv_.wait_for(lock, time_out_) == std::cv_status::timeout) { return false; } return true; }
void BreakAllWait() override { cv_.notify_all(); }
void SetTimeout(uint64_t timeout) { time_out_ = std::chrono::milliseconds(timeout); }
private: std::mutex mutex_; std::condition_variable cv_; std::chrono::milliseconds time_out_; };
|
- WaitStrategy基类:
WaitStrategy
是一个虚基类,定义了等待策略的基本接口。
- 其中包括
NotifyOne()
、 BreakAllWait()
和 EmptyWait()
纯虚函数。
- BlockWaitStrategy类:
BlockWaitStrategy
继承自 WaitStrategy
,实现了阻塞式等待策略。
- 它使用了互斥锁和条件变量,通过
cv_.wait(lock)
进行线程等待,通过 cv_.notify_one()
唤醒一个等待的线程,通过 cv_.notify_all()
唤醒所有等待的线程。
- 线程会一直阻塞在此直到另外一个线程来唤醒,当线程被唤醒后才会返回
true
- SleepWaitStrategy类:
SleepWaitStrategy
继承自 WaitStrategy
,实现了休眠式等待策略。
- 在
EmptyWait()
中,通过 std::this_thread::sleep_for()
函数使当前线程休眠一段时间。
- 线程会休眠一段时间,不会进行阻塞,睡眠时间结束后返回
true
- YieldWaitStrategy类:
YieldWaitStrategy
继承自 WaitStrategy
,实现了让出CPU时间片的等待策略。
- 在
EmptyWait()
中,通过 std::this_thread::yield()
函数让当前线程放弃其时间片。
- 线程时间片切换,返回
true
- BusySpinWaitStrategy类:
BusySpinWaitStrategy
继承自 WaitStrategy
,实现了忙等待策略。
- 在
EmptyWait()
中,直接返回 true
,表示一直忙等。
- 始终返回
true
,一直等待
- TimeoutBlockWaitStrategy类:
TimeoutBlockWaitStrategy
继承自 WaitStrategy
,实现了带有超时的阻塞等待策略。
- 它在
EmptyWait()
中,使用 cv_.wait_for(lock, time_out_)
,允许线程等待一段时间,如果超时则返回 false
,否则返回 true
。
- 线程在超时时间内没有被唤醒,此时
EmptyWait
方法将返回 false
,反映了等待过程中的超时情况。如果被唤醒,返回 true
。线程在确定时间内为阻塞状态
uml类图如下:
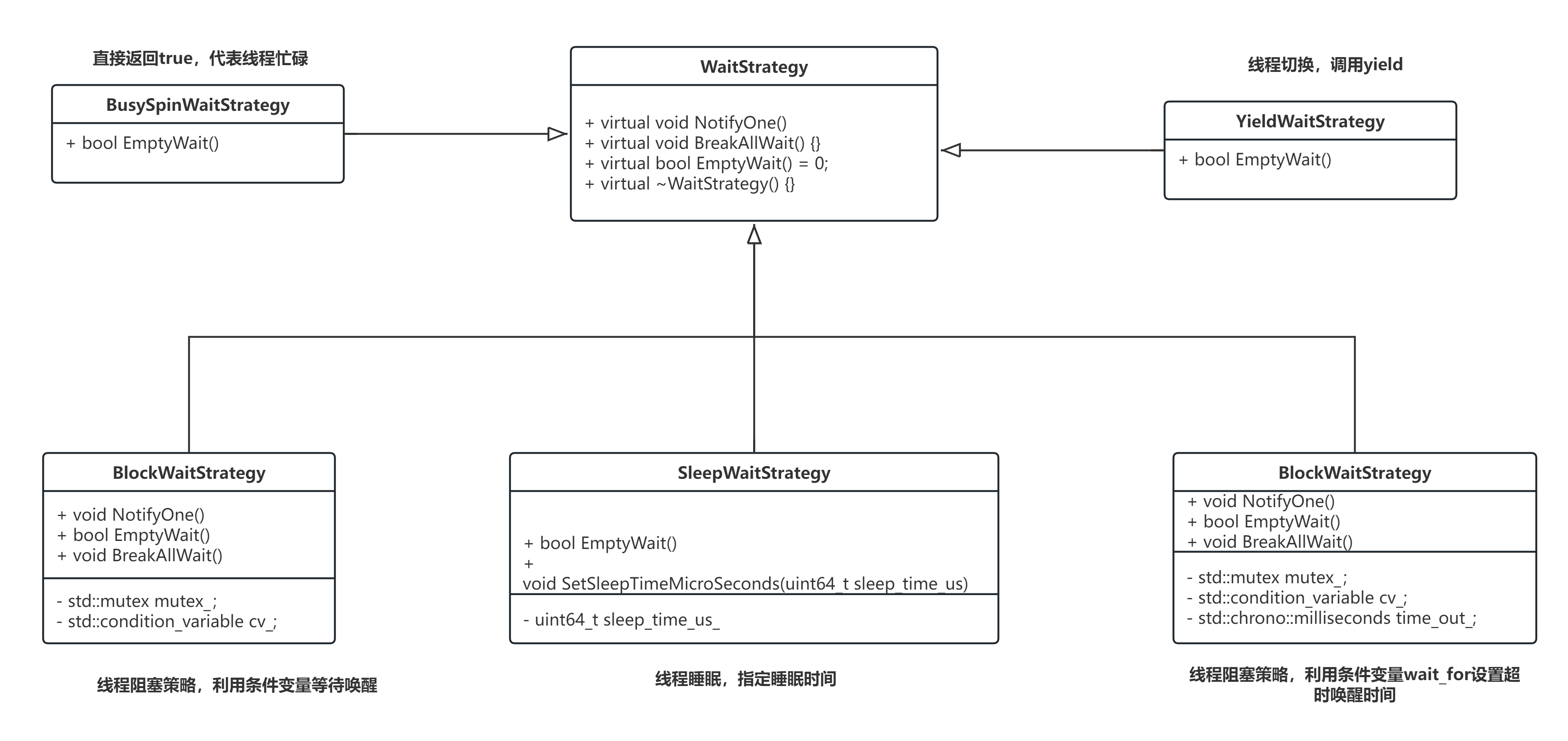
2.参考连接